Integration of Clock
- projectsilversteps
- Nov 10, 2015
- 5 min read

The DS3231 clock component has arrived in the mail. Karly connected the clock into the prototype circuit and wrote code to set the time and test to see if the clock could be read and change with time. The code used is shown below:
#include "Wire.h"
#define DS3231_I2C_ADDRESS 0x68
// Convert normal decimal numbers to binary coded decimal
byte decToBcd(byte val)
{
return( (val/10*16) + (val%10) );
}
// Convert binary coded decimal to normal decimal numbers
byte bcdToDec(byte val)
{
return( (val/16*10) + (val%16) );
}
void setup()
{
Wire.begin();
Serial.begin(9600);
// set the initial time here:
// DS3231 seconds, minutes, hours, day, date, month, year
//setDS3231time(30,4,17,3,10,11,15);
}
void setDS3231time(byte second, byte minute, byte hour, byte dayOfWeek, byte
dayOfMonth, byte month, byte year)
{
// sets time and date data to DS3231
Wire.beginTransmission(DS3231_I2C_ADDRESS);
Wire.write(0); // set next input to start at the seconds register
Wire.write(decToBcd(second)); // set seconds
Wire.write(decToBcd(minute)); // set minutes
Wire.write(decToBcd(hour)); // set hours
Wire.write(decToBcd(dayOfWeek)); // set day of week (1=Sunday, 7=Saturday)
Wire.write(decToBcd(dayOfMonth)); // set date (1 to 31)
Wire.write(decToBcd(month)); // set month
Wire.write(decToBcd(year)); // set year (0 to 99)
Wire.endTransmission();
}
void readDS3231time(byte *second,
byte *minute,
byte *hour,
byte *dayOfWeek,
byte *dayOfMonth,
byte *month,
byte *year)
{
Wire.beginTransmission(DS3231_I2C_ADDRESS);
Wire.write(0); // set DS3231 register pointer to 00h
Wire.endTransmission();
Wire.requestFrom(DS3231_I2C_ADDRESS, 7);
// request seven bytes of data from DS3231 starting from register 00h
*second = bcdToDec(Wire.read() & 0x7f);
*minute = bcdToDec(Wire.read());
*hour = bcdToDec(Wire.read() & 0x3f);
*dayOfWeek = bcdToDec(Wire.read());
*dayOfMonth = bcdToDec(Wire.read());
*month = bcdToDec(Wire.read());
*year = bcdToDec(Wire.read());
}
void displayTime()
{
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
// retrieve data from DS3231
readDS3231time(&second, &minute, &hour, &dayOfWeek, &dayOfMonth, &month,
&year);
// send it to the serial monitor
Serial.print(hour, DEC);
// convert the byte variable to a decimal number when displayed
Serial.print(":");
if (minute<10)
{
Serial.print("0");
}
Serial.print(minute, DEC);
Serial.print(":");
if (second<10)
{
Serial.print("0");
}
Serial.print(second, DEC);
Serial.print(" ");
Serial.print(dayOfMonth, DEC);
Serial.print("/");
Serial.print(month, DEC);
Serial.print("/");
Serial.print(year, DEC);
Serial.print(" Day of week: ");
switch(dayOfWeek){
case 1:
Serial.println("Sunday");
break;
case 2:
Serial.println("Monday");
break;
case 3:
Serial.println("Tuesday");
break;
case 4:
Serial.println("Wednesday");
break;
case 5:
Serial.println("Thursday");
break;
case 6:
Serial.println("Friday");
break;
case 7:
Serial.println("Saturday");
break;
}
}
void loop()
{
displayTime(); // display the real-time clock data on the Serial Monitor,
delay(1000); // every second
}
The code outputs the hour, minute, second, date, and day. Upon calibrating the clock and running the code, the clock proved to be fully functional by outputing the appropriate time and date. The image below shows the output:
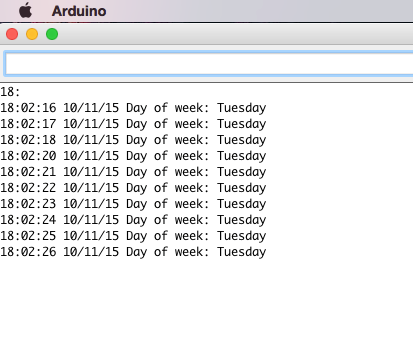
Next, Roy and Karly integrated the clock reading code into the bluetooth/accelerometer sketch. Now, when the Arduino reads the analog input pins from the accelerometer, the reading will be associated with a specific time. The time and accelerometer readings will then be transmitted to the application on our phones via bluetooth. We were able to get this system to work and the output that we got on our phones is shown below:

T
The final integrated code that includes the accelerometer, bluetooth, and clock is shown below:
/*********************************************************************
Project SilverSteps
This is code for the ADXL335 accelerometer and the nRF8001 Bluetooth module
to connect to SAMI,Samsung's development platform for its Simband.
Source code for the accelertometer:
http://www.instructables.com/id/Interfacing-ADXL335-with-ARDUINO/?ALLSTEPS
Original code for the Bluetotoh is based off of code
written by Kevin Townsend/KTOWN for Adafruit Industries.
The code has been modified to work for Project SilverSteps
*********************************************************************/
/**************************************************************************/
/*!
Initialize
*/
/**************************************************************************/
#include <SPI.h>
#include "Adafruit_BLE_UART.h"
#include "Wire.h"
#define ADAFRUITBLE_REQ 10
#define ADAFRUITBLE_RDY 2
#define ADAFRUITBLE_RST 9
#define DS3231_I2C_ADDRESS 0x68
Adafruit_BLE_UART uart = Adafruit_BLE_UART(ADAFRUITBLE_REQ, ADAFRUITBLE_RDY, ADAFRUITBLE_RST);
/**************************************************************************/
/*!
This function is called whenever select ACI events happen
*/
/**************************************************************************/
void aciCallback(aci_evt_opcode_t event)
{
switch(event)
{
case ACI_EVT_DEVICE_STARTED:
Serial.println(F("nRF8001 advertising initialized."));
Serial.println(F("Output is acceleration = (x,y,z)"));
break;
case ACI_EVT_CONNECTED:
Serial.println(F("nRF8001 module paired."));
break;
case ACI_EVT_DISCONNECTED:
Serial.println(F("nRF8001 disconnected or advertising timed out."));
break;
default:
break;
}
}
// Convert normal decimal numbers to binary coded decimal
byte decToBcd(byte val)
{
return( (val/10*16) + (val%10) );
}
// Convert binary coded decimal to normal decimal numbers
byte bcdToDec(byte val)
{
return( (val/16*10) + (val%16) );
}
/**************************************************************************/
/*!
Initialize serial communications at 9600bps and
configure the Arduino to start advertising with the radio
*/
/**************************************************************************/
//connect 3.3v to AREF
//global vars
int input[3];
double output[3];
byte second, minute, hour, dayOfWeek, dayOfMonth, month, year;
String seconds, minutes, hours, dayOfWeeks, dayOfMonths, months, years;
void setup() {
Wire.begin();
Serial.begin(9600);
// set the initial time here:
// DS3231 seconds, minutes, hours, day, date, month, year
//setDS3231time(30,4,17,3,10,11,15);
while(!Serial); // Leonardo/Micro should wait for serial init
Serial.println(F("Welcome to Project SilverSteps"));
Serial.println(F("SilverSteps Mark V loading"));
// uart.setRXcallback(rxCallback);
uart.setACIcallback(aciCallback);
// uart.setDeviceName("NEWNAME"); /* 7 characters max! */
uart.begin();
}
void setDS3231time(byte second, byte minute, byte hour, byte dayOfWeek, byte
dayOfMonth, byte month, byte year)
{
// sets time and date data to DS3231
Wire.beginTransmission(DS3231_I2C_ADDRESS);
Wire.write(0); // set next input to start at the seconds register
Wire.write(decToBcd(second)); // set seconds
Wire.write(decToBcd(minute)); // set minutes
Wire.write(decToBcd(hour)); // set hours
Wire.write(decToBcd(dayOfWeek)); // set day of week (1=Sunday, 7=Saturday)
Wire.write(decToBcd(dayOfMonth)); // set date (1 to 31)
Wire.write(decToBcd(month)); // set month
Wire.write(decToBcd(year)); // set year (0 to 99)
Wire.endTransmission();
}
//reads time and date data from DS3231
void readDS3231time(byte *second,
byte *minute,
byte *hour,
byte *dayOfWeek,
byte *dayOfMonth,
byte *month,
byte *year)
{
Wire.beginTransmission(DS3231_I2C_ADDRESS);
Wire.write(0); // set DS3231 register pointer to 00h
Wire.endTransmission();
Wire.requestFrom(DS3231_I2C_ADDRESS, 7);
// request seven bytes of data from DS3231 starting from register 00h
*second = bcdToDec(Wire.read() & 0x7f);
*minute = bcdToDec(Wire.read());
*hour = bcdToDec(Wire.read() & 0x3f);
*dayOfWeek = bcdToDec(Wire.read());
*dayOfMonth = bcdToDec(Wire.read());
*month = bcdToDec(Wire.read());
*year = bcdToDec(Wire.read());
}
/**************************************************************************/
/*!
Constantly checks for new events on the nRF8001 and maps ADXL335
*/
/**************************************************************************/
void loop() {
//acceleration
analogReference(EXTERNAL); //connect 3.3v to AREF
//input is (x,y,z) acceleration with values from 0 to 1023
input[0] = analogRead(A2);
input[1] = analogRead(A1);
input[2] = analogRead(A0);
//output maps input from -3 G's to +3 G's
output[0] = input[0] / 1023.0 * 6.0 - 3.0;
output[1] = input[1] / 1023.0 * 6.0 - 3.0;
output[2] = input[2] / 1023.0 * 6.0 - 3.0;
uart.pollACI();
//print results via Bluetooth
String out = "(" + String(output[0]) + "," + String(output[1]) + "," + String(output[2]) + ")";
uart.print(out);
//*************************************************************************//
//time
// retrieve data from DS3231
readDS3231time(&second, &minute, &hour, &dayOfWeek, &dayOfMonth, &month, &year);
hours = String(hour);
years = String(year);
if(minute<10)
{
minutes = "0" + String(minute);
}
else
{
minutes = String(minute);
}
if(second<10)
{
seconds = "0" + String(second);
}
else
{
seconds = String(second);
}
if(dayOfMonth<10)
{
dayOfMonths = "0" + String(dayOfMonth);
}
else
{
dayOfMonths = String(dayOfMonth);
}
if(month<10)
{
months = "0" + String(month);
}
else
{
months = String(month);
}
switch(dayOfWeek){
case 1:
dayOfWeeks = "Sunday";
break;
case 2:
dayOfWeeks = "Monday";
break;
case 3:
dayOfWeeks = "Tuesday";
break;
case 4:
dayOfWeeks = "Wednesday";
break;
case 5:
dayOfWeeks = "Thursday";
break;
case 6:
dayOfWeeks = "Friday";
break;
case 7:
dayOfWeeks = "Saturday";
break;
}
String out_time = hours + ":" + minutes + ":" + seconds + " " + dayOfMonths + "/" + months + "/" + years;
uart.print(out_time);
uart.print(dayOfWeeks);
delay(8);
}
Roy continued to work on the manifest that will connect our prototype board to SAMI. We are currently having issues importing the necessary objects from the SAMI library into our java code. Hence, Roy and Karly intend on meeting with Farhad to see how to resolve this issue with SAMI.
Comments